Insert data into MySQL using jQuery ajax without page refresh. Today we are going to learn how to to submit a sample form in PHP and store data into MySQL database using jQuery ajax.
Follow below step to insert data into database using PHP Ajax
Step 1 – Create Database And Table
CREATE DATABASE developer_db;
CREATE TABLE `customers` (
`id` int(10) UNSIGNED NOT NULL,
`name` varchar(255) COLLATE utf8mb4_unicode_ci NOT NULL,
`email` varchar(255) COLLATE utf8mb4_unicode_ci NOT NULL
`created_date` date DEFAULT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci;
Step 2 – Create ajax.php and insert below code
<?php
mysql_connect("localhost", "root", "") or die("cannot connect");
mysql_select_db("prodchecklist") or die("cannot select DB");
// CLIENT INFORMATION
$fname = htmlspecialchars(trim($_POST['fname']));
$lname = htmlspecialchars(trim($_POST['lname']));
$addClient = "INSERT INTO tbluser (username,userpassword) VALUES ('$fname','$lname')";
mysql_query($addClient) or die(mysql_error());
?>
Step 3 – Create index.php and insert below code
<html>
<head>
<title>
</title>
<script src="lib/jquery/jquery-1.3.2.js" type="text/javascript">
</script>
<script src="javascripts/jquery.validate.js" type="text/javascript">
</script>
<script type="text/javascript">
$(document).ready(function(){
$("form#submit").submit(function() {
$(".error").hide();
$("#loader").show();
$("#loader").fadeIn(400).html('<img src="ajax-loader.gif" align="absmiddle"> <span class="loading">Loading Comment...</span>');
var hasError = false;
// we want to store the values from the form input box, then send via ajax below
var fname = $('#fname').attr('value');
var lname = $('#lname').attr('value');
if(fname == ''){
$("#fname").after('<span class="error">Please enter a First name</span>');
$("#loader").hide();
hasError = true;
}
if(lname == ''){
$("#lname").after('<span class="error">Please enter a Last name</span>');
$("#loader").hide();
hasError = true;
}
if(hasError = false){
$.ajax({
type: "POST",
url: "ajax.php",
data: "fname="+ fname +"& lname="+ lname,
success: function(){
document.getElementById('fname').value='';
document.getElementById('lname').value='';
$("#loader").hide();
}
});
}
return false;
});
});
</script>
</head>
<body>
<div class="container">
<form id="submit" method="post">
<fieldset>
<legend>Enter Information</legend>
<label for="fname">Client First Name:</label>
<input id="fname" class="text" name="fname" size="20" type="text">
<br>
<br>
<label for="lname">Client Last Name:</label>
<input id="lname" class="text" name="lname" size="20" type="text">
<br>
<br>
<button class="button positive"> Add Client </button>
</fieldset>
</form>
<div class="success" style="display: none;">Client has been added.</div>
</div>
<div id="loader" align="left"></div>
</body>
</html>
In the above code we have created HTML and also in the same file write $.ajax which help us to call ajax.php file and do the insert data into database activity.
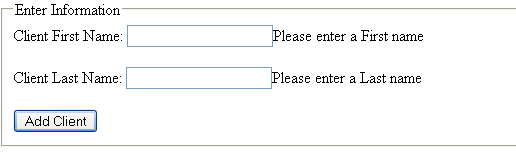