Node.js is a popular server-side Javascript platform. Too many companies use node.js year after year. When you are preparing interview for the node.js, then this article is best for you. We’ve compiled a comprehensive list of common Node.js interview questions that are commonly asked in job interviews, along with the best ways to answer them. This will also help you understand the basics of Node.js.
Top 5 Frequently Asked Laravel Interview Questions and Answers
- What is Node.js?
- What is NPM?
- How does Node.js works?
- In which Language Node Js is written?
- What are the modules in Node.js?
Q1. What is Node.js?
Node.js can be easily explained as it is a virtual machine that uses JavaScript as the scripting language and runs Chrome’s V8 JavaScript engine. In addition, Node.js is designed to build scalable network applications.
Basic Example of Nod.js Programe
var http = require('http');
http.createServer(function (req, res) {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello World!');
}).listen(3000);
Q2. What is NPM?
NPM is a package manager and command line utility that interacts with the repository and is used for installation, versioning, and also dependency management of projects. The software or packages which you want to install these applications can be searched for on https://www.npmjs.com/.
Q3. How does Node.js works?
A web server using Node.js typically has a workflow. Let’s explore this flow of operations in detail.
- Clients send requests to the webserver to interact with the web application. Requests can be non-blocking or blocking.
- Querying for data, Deleting data and Updating the data.
- Node.js retrieves the incoming requests and adds those to the Event Queue
- The requests are then routed through the event loop one at a time. Verify that the requirements are simple enough not to require external resources. The event loop handles simple requests (non-blocking operations) such as B. I/O queries, and returns responses to the appropriate clients
- A single thread from the Thread Pool is assigned to a single complex request. This thread is responsible for completing a particular blocking request by accessing external resources, such as computation, database, file system, etc.
Once the task is carried out completely, the response is sent to the Event Loop that sends that response back to the client.
Q4. How do you manage packages in your node.js project?
It is also typically monitored by various package installers and its configuration registry. Most of them use npm or yarn. Both provide pretty much all javascript libraries. To manage versions of libraries installed in a project, we use package.json and package-lock.json so there is no problem porting this app to another environment.
Q5. In which Language Node Js is written?
Node js is written in C, C++,JavaScript.It uses Google’s open source V8 Javascript Engine to convert Javascript code to C++.
Q6. How is Node.js better than other frameworks?
- Node.js offers easy development due to its non-blocking I/O, and the pair-based model results in fast response time and concurrent processing, unlike other frameworks that require developers to use thread management.
- It runs on a chrome v8 engine which is written in c++ and is highly performant with constant improvement.
- Since we will be using javascript in both frontend and backend, development will be much faster. Finally, there are many libraries, so we don’t have to worry or get bogged down in development.
Q7. What is ECMAScript?
Ecmascript first appeared in 1997, specified and developed by Esma Internationals. Evmascriot is also a programming language that has developed various other scripts such as Jscript, ActionScript and the most widely used Javascript.
Q8. What are commonly used timing featers in Node.js
- setTimeout/clearTimeout – This is used to implement delays in code execution.
- setInterval/clearInterval – This is used to run a code block multiple times.
- setImmediate/clearImmediate – Any function passed as the setImmediate() argument is a callback that’s executed in the next iteration of the event loop.
- process.nextTick – Both setImmediate and process.nextTick appear to be doing the same thing; however, you may prefer one over the other depending on your callback’s urgency.
Q9. What are the advantages of using promises instead of callbacks?
- Better defined and organized control flow of asynchronous logic.
- Highly reduced coupling.
- Integrated error handling.
- Improved readability.
Q10. How is Node.js most frequently used?
Node.js mostly used in following types of appliction
- Real-time chats
- Streaming applications
- Internet of Things
- Microservices architecture
- Real-time collaboration tools
- Complex SPAs (Single-Page Applications)
Q11. What are the modules in Node.js?
Modules are like JavaScript libraries that can be used in a Node.js application to encapsulate a set of functionality. To include a module in a Node.js application, node.js uses the require() function with parentheses containing the module name.
Below example HTTP module we used with require().
const http = require('http');
List of some modules which are commonly used in node.js application
- HTTP
- fs
- util
- url
- dotenv
- multer
- axios
- express
- cors
- body-parser
- moment
- loadash
- socket.io
Q12. What is the purpose of module.exports in node.js
In Node.js, a module encapsulates all related code into a single unit of code that can be analyzed by moving all relevant functions into a single file. You can export a module using the module and export feature, which allows you to import it into another file with a required keyword.
Q13. Which database is more popularly used with Node.js?
MongoDB is the most common database used with Node.js. It is a NoSQL, cross-platform, document-oriented database that provides high performance, high availability, and easy scalability.
Q14. What are the pros and cons of Node.js?
Pros | Cons |
---|---|
Fast processing and an event-based model | Not suitable for heavy computational tasks |
Node Package Manager has over 45k+ packages that provide the functionality to an application | Dealing with relational databases is not a good option for Node.js |
Best suited for streaming huge amounts of data and I/O intensive operations | Since Node.js is single-threaded, CPU intensive tasks are not its strong suit |
Q15. What is an Event Loop in Node.js?
Event loops handle asynchronous callbacks in Node.js. It is the basis for non-blocking I/O in Node.js, making it one of the most important environment features.
Q16. Is Node Js Single-threaded?
Node Js is single-threaded but also non-blocking, which means that while Node Js is running, various functions like callbacks are running in the background, which is run with different threading or multi-threading.
Q17. Differentiate between process.nextTick() and setImmediate()?
The difference between process.nextTick() and setImmediate(). This is accomplished through the use of nextTick() and setImmediate(). next Tick() postpones the execution of action until the next pass around the event loop, or it simply calls the callback function once the event loop’s current execution is complete, whereas setImmediate() executes a callback on the next cycle of the event loop and returns control to the event loop for any I/O operations.
Q18. How many types of API functions are there in Node.js?
Asynchronous, non-blocking functions – mostly I/O operations which can be fork out of the main loop.
Synchronous, blocking functions – mostly operations that influence the process running in the main loop.
Q19. What is the package.json file?
The package.json file is the heart of a Node.js system. This file contains the metadata for a specific project. The package.json is located in the root of each Node application or module
This is what a package.json file looks like immediately after creating a Node.js project using the command: npm init
You can edit the parameters when you create a Node.js project.
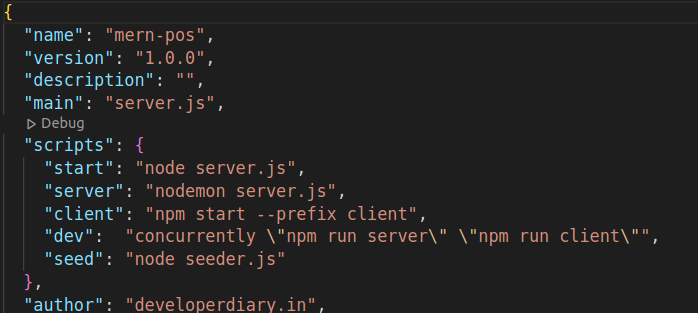
Q20. What is the Express.js package?
Express is a node js web application framework that provides broad features for building web and mobile applications. It is used to build a single page, multipage, and hybrid web application.
Q21. How do you create a simple Express.js application?
Following is the simple code on express.js.
const express = require('express')
const app = express()
app.get('/', (req, res) => {
res.send('Hello World!')
})
app.listen(3000, () => {
console.log(`Example app listening on port ${port}`)
})
You can access this app using http://localhost:3000.
Q22. What are streams in Node.js?
Streams are objects that enable you to read data or write data continuously. There are four types of streams:
- Readable – Used for reading operations
- Writable − Used for write operations
- Duplex − Can be used for both reading and write operations
- Transform − A type of duplex stream where the output is computed based on input
Q23. What are the different types of HTTP requests?
There are following types of http requests
- GET: Used to retrieve the data
- POST: Generally used to make a change in state or reactions on the server
- HEAD: Similar to the GET method, but asks for the response without the response body
- DELETE: Used to delete the predetermined resource
You can read more about get and post methods
Q24. How we can connect MongoDB with Nod.js
The MongoDB module exports MongoClient, and that’s what we’ll use to connect to a MongoDB database.
const {MongoClient} = require('mongodb');
const uri = "mongodb+srv://<username>:<password>@<your-cluster-url>/test?retryWrites=true&w=majority";
const client = new MongoClient(uri);
try {
// Connect to the MongoDB cluster
await client.connect();
} catch (e) {
console.error(e);
} finally {
await client.close();
}
Q25. What is REPL?
REPL – Read, Eval, Print, Loop.
It is an environment for input commands to perform REPL tasks. Each of the tasks is associated with its respective operations.
What Is UseQuery (React Query) With Example
What Is UseState Hook In React With Example
More Interview Questions
PHP OOPS Interview Questions And Answers (2022)
Latest MySQL Interview Questions And Answers
JavaScript Interview Questions And Answers
CodeIgniter Interview Questions And Answers
PHP Interview Questions And Answers
Laravel Interview Questions And Answers
Conclusion
I think these Node.js interview questions would help you understand what kind of questions you might be asked in an interview and by going through these Node.js interview questions you can prime and crack your next interview in one fell swoop. And I’ll try to keep updating the interview questions here. So you can dig deeper.