Now a days react coming with hooks functions. We already learn about useState, useEffect hooks in our previous article. Today we are going to learn about useContext hooks. This hook is also very popular in react programming.
What is useContext?
In simple definition react context is use to handle state globally without using or pass any props in the components. Lets understand why and how we can use “useContext()” hook in our program.
props Problem
When you use props and need to pass data to the last child component among multiple nested components. Data must be passed through each component in the tree using props. Therefore, each component must know this accessory data. even if you don’t use it.
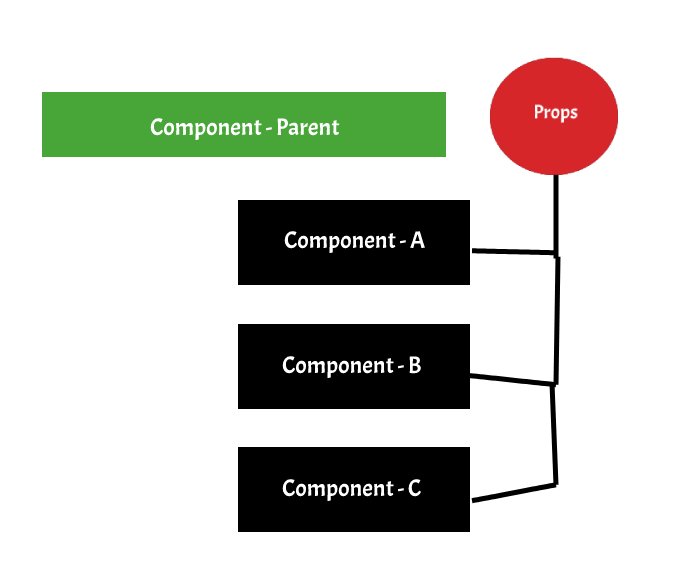
useContext Solve props Problem
At the top you can set the context and all the components in the middle don’t have to know anything about it and the bottom one can access it. In a way, it fulfills the same requirements as Redux.
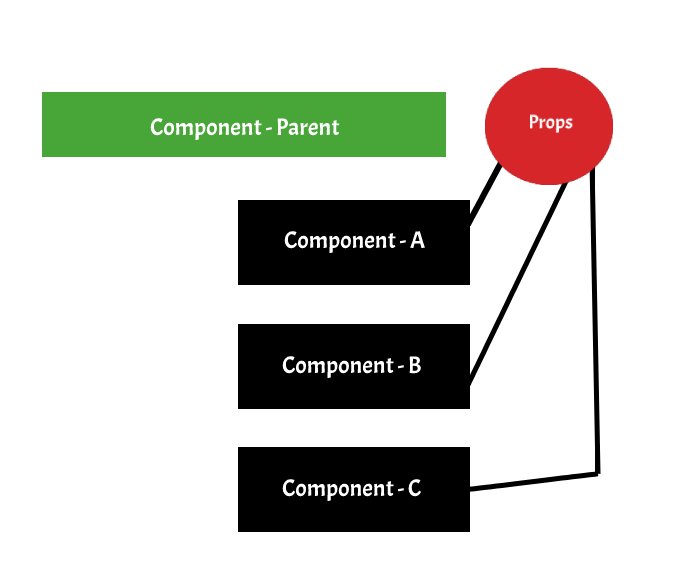
So the useContext hook helps make the code more readable and less verbose, and eliminates the need to introduce the consumer component. The useContext hook is the new addition in React 16.
Syntax
const nameContext = useContext(initialValue);
useContext accepts the value provided by createContext and re-renders the component each time its value changes, but you can still optimize its performance by using memoization. The idea of using the context is to allow components to access global data and re-render it when that global data changes. Context solves the prop piercing problem when you need to pass props from parent to child.
In below example we are creating first context and initialize with value
import { useState, createContext } from "react";
const UserContext = createContext();
Using Context Provider wrap child components in the Context Provider also supply the state value with the provider. As per my requirement I am wrapping ComponentOne
<UserContext.Provider value={user}>
<h1>{`Hello ${user}!`}</h1>
<ComponentOne />
</UserContext.Provider>
Next and last step import the useContext in ComponentFour where you want the parent state value without passing any props.
import { useContext } from "react";
Following is the full example where you will understand the useContext which I already explain above. In the below example we create Five component componentOne, componentTwo, componentThree, componentFour and parent component is App.js. Here you will see we are accessing value in componentFour.js without passing any value in all component via props.
useContext Full Example
App.js
import { useState, createContext } from "react";
import ComponentOne from './ComponentOne.js';
const UserContext = createContext();
export function App() {
const [user, setUser] = useState("Developer Diary");
return (
<UserContext.Provider value={user}>
<h1>{`Hello ${user}!`}</h1>
<ComponentOne />
</UserContext.Provider>
);
}
ComponentOne.js
import ComponentTwo from './ComponentTwo.js';
export function ComponentOne() {
return (
<>
<h1>Component One</h1>
<ComponentTwo />
</>
);
}
ComponentTwo.js
import ComponentThree from './ComponentThree.js';
export function ComponentTwo() {
return (
<>
<h1>Component Two</h1>
<ComponentThree />
</>
);
}
ComponentThree.js
import ComponentFour from './ComponentFour.js';
export function ComponentThree() {
return (
<>
<h1>Component Three</h1>
<ComponentFour />
</>
);
}
ComponentFour.js
import { useContext } from "react";
export function ComponentFour() {
const user = useContext(UserContext);
return (
<>
<h1>Component Four</h1>
<h2>{`Hello ${user}!`}</h2>
</>
);
}
Output
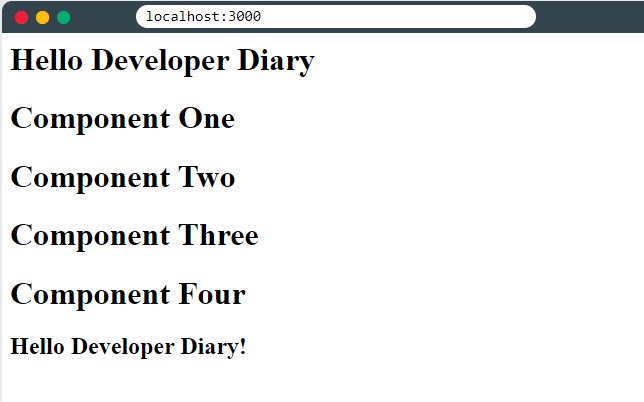
Others ReactJs Hooks
Conclusion
In this article we understand the useContext. Hope you all understand the what is useContext hooks. In next article we will understand next hooks.