In this post we’ll cover what is useRef Hook in React. The useRef hook is new in React 16.8. Before proceeding with this post, you need to know the ref in React. The useRef hook is a hook that allows you to directly create a reference to the DOM element in the functional component.
The useRef
Hook allows you to persevere values between renders.
const refContainer = useRef(initialValue);
useRef returns a mutable reference object. This object has a property called .current. The value is held in the refContainer.current property.
These values are accessed through the current property of the returned object. The .current property could be initialized with the passed argument initialValue e.g. useRef(initialValue). The object can retain a value for the lifetime of the component.
useRef Example
import React, { useRef } from "react";
export const TextInputExample = () => {
const textInput = useRef();
focusTextInput = () => textInput.current.focus();
return (
<>
<input type="text" ref={textInput} />
<button onClick={focusTextInput}>Focus input</button>
</>
);
}
When you click Focus input button as per above code. You curser auto focus on input box using useRef hook
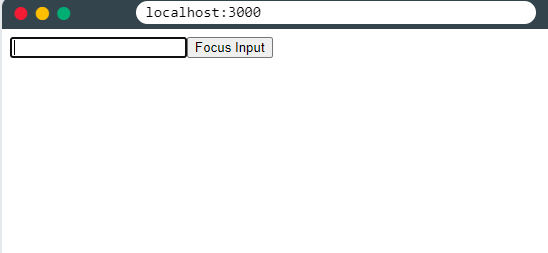
Note: For a functional component, we use useRef instead of createRef. When you create a reference with createRef in a functional component, React creates a new instance of the reference with each render instead of keeping it between renders.
Using useRef we can track the previous state values. This is the reason we are able to persist the state value between the renders.
Track State Example
import { useState, useEffect, useRef } from "react";
import ReactDOM from "react-dom/client";
export function TrackState() {
const [inputValue, setInputValue] = useState("");
const previousInputValue = useRef("");
useEffect(() => {
previousInputValue.current = inputValue;
}, [inputValue]);
return (
<>
<input
type="text"
value={inputValue}
onChange={(e) => setInputValue(e.target.value)}
/>
<h2>Current Value: {inputValue}</h2>
<h2>Previous Value: {previousInputValue.current}</h2>
</>
);
}
In the above example we use useState, useEffect, and useRef to keep track of the previous state values.
As you see in the useEffect function we are updating the useRef value means current value each time the inputValue is updated when we enter a text into the input field.
Related Articles
What Is UseQuery (React Query) With Example
What Is UseState Hook In React With Example
Conclusion
At a first look it may be little hard to understand. Don’t rush it! If you’re lost in the explanation, look at the code above again and try to read it from start to end and also try to run program on your computer. I promise if you do this you don’t forget about react useRef() hook. Must try it.